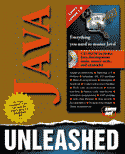
Chapter 37
The bane of any software developers existence is embodied by a seemingly innocuous word: documentation. The old coders war cry, If it was hard to write, it should be hard to read and use, has no doubt been heard by some readers. Part of this general malaise can be attributed to the sometimes disjointed methods by which software documentation is createdan awkward cycle of creating code, commenting, extracting comments (if any in the first place), formatting in perhaps a word-processing package, preparing, and finally distributing it internally.
However, on some operating systems such as UNIX, editors exist that can be taught to perform such tasks (for example, the ubiquitous Emacs). While useful, such an approach always is parochial. There are no defined standards for commenting C++, C, or even Smalltalk code in a universally understood manner. Code documentation generally remains a weak point of the overall development process, because any code base that requires no maintenance probably is not useful anymore, and any maintenance effort is hampered by poor, incomplete, or missing documentation.
The developers of Java, however, decided to address such issues. It is possible with Java to add code commentary and produce documentation simultaneously and painlessly. Additionally, documentation can be released with Java products, eliminating the time lag between software and its accompanying explanatory text. The Java API documentation is itself generated from the Java class library source code and is copiously hypertext-linked, indexed, and presented attractively. With Java, it might even be possible, in some small way, to actually enjoy this process! We shall explore some of the rationale behind good documentation, the Java tools available for documentation, and metrics that can be used to measure what is finally produced in this chapter.
As mentioned, documentation still is a relatively thorny and unpopular issue. In an attempt to soothe any qualms you might have, we are going to lay out some reasons why you should document your code, and document it well.
Five Reasons to Document
There are more than five reasons to document, but here is a selection of some of the more compelling ones:
- Think of the next person.
- phrase to consider: staff turnover. You may already have had this happen to you, but imagine the frustration of encountering a large body of code that has no comments. What will be the first task you have to perform on code like this? No doubt it will be to find an obscure bug that has manifested itself after the last developer left. If programmers could be confident that any piece of software they create never will require adjustment, the documentation issue is unimportant. Unfortunately, it would take more years than man has been on the Earth to prove mathematically that a piece of software is correct, so documentation remains important.
- Your code can be an educational tool.
- value of a well-designed, well-constructed, and well-documented piece of software cannot be underestimated as an educational aid. However, given the complexity of some of the tasks programmers are called on to perform, design and implementation decisions that are made might appear obscure without commentary. Good documentation supports leveraging of intellectual effort.
- No language is self-documenting.
- phrase, the language is self-documenting, used to be heard to justify the lack of code comments and explanatory text. If only this were true, but we know differently. Some features of even Java could need to be highlighted for less-experienced developers, including perhaps some in your own organization. An example of this highlighting might be to state in a comment that the implementation of an abstract function is the responsibility of a subclass (that is, a derived class). This fact might seem obvious after a few days, weeks, or months with Java, but it can mean an awful lot to someone just getting started.
- You can comply with Java coding standards.
- class, method, parameter, and variable comments might just form part of a coding standard that you follow, or might wish to follow. This is not to say that draconian measures are called forjust some standards that fall into the general area of making your life easier. Some suggested standards are described later on.
- You can automate its production.
-
Java, by utilizing the javadoc utility it is possible to create slick HTML documentation with hypertext links and inline images by adhering to the defined javadoc markup. The markup is the collection of tokens that partially represent the Java API structure. These tokens are optional text tags that allow this generation to be performed. One supposes that it would be possible to integrate such documentation into the language itself. Java is still youngthere is time.
Guidelines for Successful Java Documentation
Because you made it this far, we hope that you might also be interested in some guidelines that have proven useful empirically over the course of a number of mostly object-oriented projects. These guidelines, however, are for the most part paradigm- and language-independent. They are offered to augment the basic Java facilities for documentation.
- Copyright statement
- you are publishing your code on your web server, a public site such as Gamelan, or just allowing people to access it using anonymous FTP, be sure to include a copyright statement. Copyright statements should include items such as the conditions to impose on users of your software and what kind of warranty you do or do not imply. Pro forma text might be, <person/organization/I> hereby release the software into the public domain, and assume or assert no ownership or copyright over said software, permit free and unhindered source code modification and distribution, completely releasing all intellectual and commercial rights, and so on. A more conservative approach would be to allow it to be distributed and reused freely as long as the code derived from your code carries an acknowledgment. This is certainly a more prevalent approach and one hopes that, by and large, it is honored.
- Modification history
- you are using some form of version control system, you might already have this in place and can easily integrate it with your existing code base. However, if you are not using a tool, it might be germane to include a modification history, in chronological order, for maintenance purposes. Such a history should probably mention the author, date, and a brief but complete description of the changes, additions, and deletions. Because we primarily are interested in javadoc, you also can include some HTML markup tags if desired to spice up the details. Remember not to include items such as line rulers or anchors in your comments, though.
- javadoc markup
- covered before, because you can simplify the code, comment, and documentation phase with an automatic generator, you should! Java markup is relatively simple and self-consistent, so it presents no barrier to implementation. When writing comments, all you have to do is add a few additional characters and you are there. javadoc itself manages the more complex tasks of rendering a textual representation of the inheritance trees of your classes, the ordering of private, protected, and public members, indexes for constructors and member functions, and many others. Documentation this easy cannot be ignored!
- Looking ahead
- you are not using a version control tool for your source management, you ought to consider using one of the freely available products such as SCCS (Source Code Control System), RCS (Revision Control System), or CVCS (Concurrent Version Control System). To retrieve these, you can start your search on http://www.yahoo.com and go from there (search for RCS or GNU, perhaps). The details of such packages are beyond the scope of this chapter, but by including their markup as well as javadocs, you can integrate the two. For example, RCS has keywords such as $Id$ that it will convert to a date, version, time, and so on when you update your source to the RCS repository (a holding pen for master copies of source code and history). If you combine this with the javadoc @version tag covered later, you can have your version control tool fill in the version field for you as a by-product of good source management.
- Coding standards
-
could devote many pages to a consistent and semantically rich coding standards scheme, but will content ourselves with an aspect of coding practice that can increase your code comprehension rate and that of your colleagues: variable naming conventions. These include Hungarian notation, no notation, and various other schemes. The table below shows a simple system that is straightforward, concise, and quick to implement.
Table 37.1. Sample variable naming scheme for Java.
Variable Prefix | Meaning | Example |
I | Integer variable | iNumberOfEvents |
l | Long integer | lNumberOfEvents |
f | Floating-point variable | fNumberOfStars |
d | Double variable | dNumberOfNebulae |
c | Character | cSmallCharacter |
s | String or string buffer | sLabel |
t | Thread | tAnimationThread |
r | Reference to variable (all variables in Java) | rMemberFunction |
x | An exception | xIoException |
b | Boolean | bMorePages |
The javadoc Utility
The thrust of this chapter is to encourage the use of the javadoc utility as a useful aid during software development. This section covers the use of HTML as a help system, navigating the generated documentation such as the Java API documents, the tags used to mark up code, and the command-line usage of javadoc itself.
HTML as a Help System
Although it seems the obvious choice as a supporting help system for a web programming language, HTML actually offers certain advantages as an API help system. Some are obvious, such as general formatting, graphics inclusion, hypertext linking, and the ability to link to remote sites through the general web structure. Other less obvious advantages include portability, symmetry with one of the intentions of Java (architectural neutrality), and the ability to recite the whole document structure without comprising the links therein (in the case of javadoc-generated documentation). The advantage of portability is sort of obvious, but you can see its value when trying to use an RTF or Windows help file under Solaris.
Navigating the javadoc-Generated Documentation
The HTML documentation that composes the Java API was generated using the javadoc utility with a few exceptions edited by hand that will be discussed later. A discussion of the underlying structure of these documentsa separately obtainable part of the Java Development Kitand their navigation covers both the online documentation and your own documentation in one fell swoop. In the text that follows, <directory> represents the base directory into which you uncompressed the API documentation. After a little while, you may begin to wonder why there is no applet content in the generated pagesas it would not have been difficult to include it.
API Users Guide Entry Screen
Figure 37.1 shows the first screen that you will see when perusing the Java API Users Guide.
FIGURE 37.1.
The opening screen for the API User's Guide.
Figure 37.1 is the file <directory>/api/API_users_guide.html, the HTML document that is the entryway to the users guide. Click the top hypertext link, Java API, to progress to the next page, as shown in Figure 37.2.
FIGURE 37.2.
The package index document, showing the applet packages and others.
This file, located in <directory>/api/Packages.html, has been manually edited post production. Each package as listed corresponds to those present in the java.* and sun.tools.debug packages. Each of these packages is a hypertext link to the classes, interfaces, exceptions, and errors of that package. So, by clicking the java.awt link, you would see Figure 37.3.
FIGURE 37.3.
The java.awt package API document.
Figure 37.3, <directory>/api/Package-java.awt.html), contains a number of interesting navigational features. As you can see, the document is partitioned into a number of indexes, asfollows:
- Interface: All links listed here will jump to interfaces defined in this package.
- Class: Hypertext jumps to the individual classes are shown here.
- Exception: The very important exceptions category, where exceptions thrown by classes in this package are detailed.
-
Error: A special category of throwable objects.
At the top of the document, you will see the following links:
- All Packages: Returns you to the document shown in Figure 37.1, displaying all of the packages available for perusal.
- Class Hierarchy: Invokes the display of the document <directory>/api/tree.html, which is a hypertext list of the Java class hierarchy, including the package that you are examining. You will see something similar to Figure 37.4.
FIGURE 37.4.
Class hierarchy document tree.html.
-
Index: Displays the file <directory>/api/AllNames.html, which is an alphabetical index allowing searches using the initial character of a variable, method, interface, exception, class, or package. Entries in the list are hypertext-linked to the corresponding explanatory documents. Figure 37.5 shows the top of the index for the Java API documentation.
FIGURE 37.5.
Index document AllNames.html.
Class Document
Each class in a package will have its documentation present in a file called <fully qualified class name>.html, where <fully qualified class name> denotes a full package name specification. Each file has a common structure consisting of the following:
- Class name: Fully qualified class name.
- Class hierarchy: Preformatted inheritance tree for the class. Each superclass is a hypertext link to the corresponding details.
- Class Details: A textual description of the current class, including its immediate superclass and what interfaces it implements, if any.
- Class comment: The descriptive class comment.
- Indexes: The variable, constructor, and method indexes. A one sentence description follows the index entry. For variable indices, types are excluded. For methods, return type is excluded. For both methods and constructors, parameter types only are listed. Each abridged entry in the index is a hypertext link to the expanded description.
-
Descriptions: Each particular entity (variable, constructor, method) has a different possible documentation display. For more details on this, see Table 37.2. The method descriptions, apart from any comments or tags that have been defined, also might include a hypertext entry to a method that is being overridden by this method. This could be of assistance in a number of situations, including the determination of the rationale for doing so and checking design decisions.
To also aid in navigation, the top of the document has hypertext links that enable you to jump to the following:
- the All Packages document
- Class Hierarchy
- the contents of the package of this class
- the previous and next class, interface, exception, or error in the package, determined alphabetically within each type of package component
-
the Index document
All of these document types have been covered in previous sections. Figure 37.6 shows the top of the java.awt.Panel class page.
FIGURE 37.6.
The AWT Panel class help page, java.awt.Panel.html.
javadoc Markup
We have mentioned already that documentation generation is achieved by executing the javadoc utility. However, we have only briefly touched upon the fact that a tag-marking scheme is required for source code files to be suitable candidates for parsing. This section covers all of the details regarding this text markup, and reiterates some of the ways in which you can enhance the basic javadoc scheme.
NOTE |
Even though javadoc will generate linked and attractive documentation, it is not a substitute for well-written comments. |
The command-line usage of javadoc is covered later.
What Documentation javadoc Will Generate
Documentation can be generated for the following:
- Packages
- Classes
- Interfaces
- Exceptions
- Methods
-
Variables
Note that only text will be generated for public and protected aspects of objects. This is in accordance with the basic object-oriented principle of encapsulationa human API browser should not be aware necessarily of the internals of class, for example. Do not take this as a method to avoid documenting implementations, though!
Embedding HTML Sequences
Virtually any of the standard HTML tags can be embedded inside source text for eventual inclusion into the generated documents. Common sense dictates (as does Sun Microsystems) that formatting tags, anchors, lists, horizontal rulers, and other tags might interfere with the documents that are produced by javadoc. It is a reasonably good way of adding some extra information in a visually striking way, thoughsuch things are best determined empirically.
Available Tags
The following table defines each currently available tag, its scope, and associated semantics. Note that they are only significant when enclosed by the special comment-opening characters /** and closing characters */ (like a fancy C comment, really). The semantics are expanded later along with some code examples. (Note: The letters fq in the following table are an acronym for fully qualified.)
Table 37.2. Defined javadoc markup tags.
Tag/string | Scope | Semantics |
@see classname | Class | See also hypertext link to the class specified |
@see fq-classname | Class | Hyperlinked see also entry |
@see fq-classname#method-name | Class | Hyperlinked see also entry to the method in the given class |
@version version text | Class | Version entry |
@author author | Class | Creates an Author entry |
@see classname | Variable | See also hypertext link to the named class |
@see fq-classname | Variable | Hyperlinked see also entry to the given class |
@see fq-classname#method-name | Variable | Hyperlinked see also entry to the method in the named class |
@param parameter-name description | Method | Parameter specification for the Parameters section |
@return description | Method | Returns section, specifying the return value |
@exception fq-class-name description | Method | Throws entry, which contains the name of the exception that might be thrown by the method |
There are some conditions that apply to the use of tags, and they are as follows:
- Class names: javadoc will prepend the name of the current package to a bare class name if the source itself is resident in a package. There capability also exists to fully qualify class names if its necessary to reference a class in a package other than the current one.
- Tag grouping: Repeated tags must be sequentially ordered. Keep all like tags together or javadoc will become confused.
- Format: All tags are prepended with the @ character and are placed at the beginning of a comment line. Leading white space and asterisks are ignored when the tag is searched for. The examples later show this clearly.
-
Length: All comments can be spread over a number of lines. In fact, a one-line comment (certainly for class scope) is too short. Have you anything else to say to enlighten your reader?
Class Comment Example
A class comment is arguably the most important you can make! Be sure to include a general description of the intent, general behavior, version, authorsperhaps including some of the look ahead tokens for version control, date, and any reference to related classes. Listing 37.1 shows a rather short class comment.
- Listing 37.1. Example class comment.
//=================================================================================
/**
* This class is part of a subsystem to parse a configurable grammar token stream
* using various recovery schemes, as detailed in the specification, under section
* 2.3, Finite State Machine recovery. An internally-mounted Web page
* <A HREF=http://ow:8080/specifications/parser/fsmrecov2.3>details this</a>.
* <br>
* @see org.parser.fsm for the finite state machine details
* @see java.io.InputStrean for the protocol our token stream adheres to
* @author T Beveridge
* @Author Natasha Brown
* @version 12/10/95, 1.00
*/
//=================================================================================
class StackedTokenStream extends org.tokenStream implements Runnable {
Note the placement of comment lines and text. There also are some extra features here, in that we have included our own hypertext reference to a specification document on our internal web server. The use of the <br> tag also inserts an empty line for neatness. Multiple tags have been used to reflect the two authors of the class, and our version details will show as a date followed by our own version number.
Method Comment Example
Remember that only public or protected methods will have documentation created. It is, of course, your decision whether to include comments for private methods, but for all except the most trivial (such as accessors), it is good software engineering practice to include comments.
TIP |
javadoc takes the first sentence of a method comment and uses it for the index entry. Therefore, it is a good idea to make this sentence descriptive. For a constructor, rather than having, Constructor. Taking X arguments
, use, Constructor taking X arguments,
. |
Describing parameters is helpful in Java, because there is no syntactic means of ascribing the C++ concept of ascribing state constancy to a parameter; this even though some parameters might never be modified when used by the method. The description of exceptions also is excellent practice because they will either have to be caught by your clients code, caught and re-thrown, or passed on. Listing 37.2 illustrates a helpful method comment.
- Listing 37.2. Example method comment.
//=================================================================================
/**
* This method determines if the Finite State Machine is in one of the 2
* (desirable) states given. The StringBuffer parameter is the only one of the 3
* that may change during the execution of this call. Note that this method may
* become private, so it may be a good idea to avoid it ! <br>
* Has not been modified for some considerable time so could be considered stable
* (or obsolete).<br> @param rStateOne An instance of FsmState. The order is
* irrelevant @param rStateTwo An instance of FsmState. The order is irrelevant
* @param rStrBuffer <b>volatile</b> This wil be updated during this call with the
* state actually encountered if this function returns true
* @return true if one of the two states has been encountered ; false (!) otherwise
* @exception java.io.IOException Thrown when the Fsm delegator attempts to fetch a
* token and fails. Unusual
* @exception org.exceptions.FsmDead Thrown when the Finite State Machine is in a
* GCablestate.
*/
//=================================================================================
protected boolean duplexState(FsmState rStateOne, FsmState rStateTwo, StringBuffer ÂrStrBuffer) {
if (bTerminated)
throw new org.exceptions.FsmDead(FSM: Dead state entered);
Again, note the inclusion of <br> tags to introduce blank lines and the use of a boldface volatile comment on the rStrBuffer description to indicate its potential value change.
Variable Comment Example
Because only the comments of protected and public variables are candidates for documentation inclusion, you might not see many of these. They seem to appear mainly for FINAL variables, which are really just a Java method for introducing constant values. Remember that variable comments for javadoc can only contain @see directives. Listing 37.3 shows a sample variable comment.
- Listing 37.3. Sample variable comment.
//=================================================================================
/**
* This boolean variable holds our live/dead status. It is held to be true through
* out the useful life of the Finite State Machine, being rendered false only when
* the associated token stream is in error or throws an exception.
* @see org.io.streams.StackedTokenStream
*/
//=================================================================================
protected boolean bTerminated;
Generating Documentation
Now we cut to the chase. We will discuss how to generate your documentation, where it can reside, platform differences, and errors that can occur.
- Where do documents go on creation?
- go wherever you happen to be, actually. What this means is best explained with an example. If you are in directory X, and you generate documentation for your package org.utilities, javadoc will dump its output in directory X. This is not particularly unfriendly unless you have manually edited Packages.htmlif you have, the javadoc process will overwrite your changes! The moral of this example is that javadoc is essentially a batch utility if you dont want to change directories too often. There also is a command-line option you can supply to alter this behavior.
- Merging your own packages with the Java API documentation
- is entirely possible though not necessarily advisable. All you really have to do is edit the Packages.html file and insert your own hypertext links to your package documents. Note that merging your own documents this way will not include them in either the index or class hierarchy.
- Platform differences
- depends very much upon your own system setup. For example, suppose you are using Windows NT javadoc to generate documents and these documents are targeted to be stored on a network drive whose native type is UNIX. This will generate documents that can be accessed under NT but not under a UNIX-based browser. Why is this? Case sensitivity. Generating from NT, javadoc seems to ignore case and the result is hypertext-linked documents that show up as errors under UNIX. This is a minor point, but if you are batch-creating documentation for a large package or number of packages, it might save you some time. In this sort of environment, maybe you should just go with UNIX-based generation!
- Other errors
-
out for the command-line syntax. You must specify a full package name, and the source must be available and readable. Any other course of action equals frustration. Make sure that you can write in the directory you are currently in or are directing the javadoc HTML documents to.
javadoc: Command-Line Usage
javadoc is just about the simplest utility in the JDK. The syntax is as follows:
javaDoc [options] {Package Name or File Name}.........
Available options are as follows:
-d <directory>
This instructs javadoc to redirect output to <directory>. The default is the current directory.
-classpath
This allows the specification of a classpath. It is the same as javac and overrides the CLASSPATH environment variable.
-verbose
This instructs javadoc to print a list of files loaded during execution. From JDK Beta 2, this might include files loaded from a ZIP file.
Measuring Your Documentation Quality
javadoc certainly is a tremendous help when it comes to automating the sometimes arduous task of creating software documentation. One aspect it does not cover is that of quality. This section attempts to provide some heuristics that can be applied to determine if the documents you are producing are worthy of a readership. Please note that a subset of this information also is included in the book Object Oriented Software Metrics by Mark Lorenz and Jeff Kidd, which is a recommended read for those interested in object-oriented metrics.
The list that follows presents metrics that you might consider adopting:
- Size/existence of class comment
- the object was important enough to warrant a class, it certainly has a purpose, provides some service, collaborates with others, or acts autonomously. Otherwise you probably would not have bothered, so it therefore deserves a commentand not just one that mentions the date and version, either! As an API reader, you should be able to read the overall class comment and either discard the class from consideration or investigate further. Without a class comment of some depth, such a task is rendered more difficult. At least 50 words would be required for a small class, such as a support class.
- Size/existence of method comments
- for similar reasons to the above, a method should be commented if it is regarded as central to the public or protected behavior of a class. Implementation methods are arguably more important to comment, but rememberthey wont be visible to your readers. Around 20 to 25 words is a good baseline for method comments.
- Number of methods/classes/interfaces/variables with comments
-
public or protected methods, classes and others, a coverage of less than 80 percent should be a cause for concern. Unless you are creating a popular class such as a string, for example, its use might not necessarily jump out at your audience. Comment it and they might just want to use it.
Currently, there is no way in Java to automate this process of documentation-quality measurement. However, if you avail yourself of the Beta 2 source code and adhere to the source license agreement, it would be possible to modify the javadoc source code to incorporate such abilities. This will at least give you timely statistics on quality automatically if you require them. The javadoc source code itself is in the package java.tools.javadoc.
This summary presents some possible improvements in javadocthough not exhaustivelyand a final endorsement of its use.
Potential Improvements
As with all tools, there always are improvements that could be made. Some of the more interesting ones include the following:
- Package comments
- is no facility currently for package comments.
- Incremental updates
- allow the documentation for just a particular class to be generated in the target directory without rewriting some of the reference files.
- Tag granularity
- example, it is possible only to provide authors on a class basis. It sometimes is desirable to note authors of methods, too, in a consistent fashion.
- Object Oriented Analysis/Object Oriented Design concepts tags
- would be reasonably useful to provide some way of noting design decisions in a way that is separate from the rest of the documentation. An example might be that a particular class is tightly coupled with certain other classes, or that it might be only ever part of an aggregation.
- Metrics
- is no provision for integration or production of metric information regarding quality.
- Liven up the documents
-
one of the objectives of Java is to make Web pages come alive, some applets that augment the utility of the API documents would be good!
This chapter has shown that javadoc is a simple and reasonably effective tool for generating API documentation quickly and effectively. Used properly, it can create useful documents to aid the users of packages. It is recommended.